This course will be retired on July 14, 2025.
Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player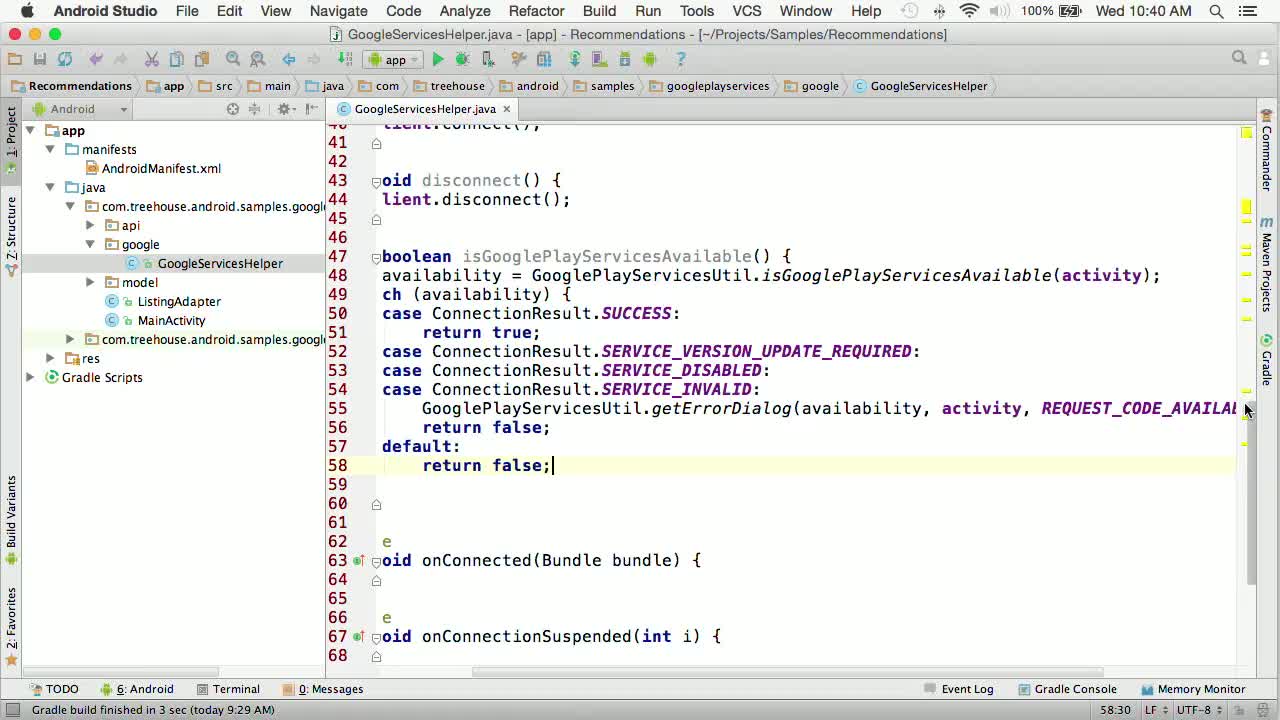
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Add in the simple cases for callbacks using the listener's onConnected() method.
This video doesn't have any notes.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
Okay, now that we have this method we've
created, isGooglePlayServicesAvailable,
0:00
let's go ahead and use it when we're
trying to connect and disconnect.
0:04
So in these cases,
we're going to just check.
0:08
If it's available, we want to connect,
and if it's not available,
0:10
well, what we're gonna do is, if for
some reason it was already connected,
0:15
we can just handle this by saying, okay,
listener, you're disconnected now.
0:20
We weren't actually connected, but
for the listener, the listener,
0:25
remember, is the activity
display in the UI.
0:29
All they care about is it's not available.
0:31
And the onDisconnected is the method
we're gonna use to inform the activity or
0:33
adapter, whatever is listening,
0:39
to know that Google Play
services is not available.
0:40
Here we're gonna also check
if it's not available, and
0:44
if it is available,
we want to go ahead and disconnect.
0:49
Otherwise, we're gonna do
the same thing and just say okay,
0:53
hey, listener you're disconnected.
0:55
Google Play services is not available,
you need to go ahead and
0:58
handle that situation.
1:01
All right.
This looks good.
1:03
Let's go ahead and keep going.
1:04
We need to fill in a few
more methods here.
1:05
We have onConnected.
1:07
So, this is a great case.
1:09
This is, we've connected.
1:11
We're successful, so
let's tell the user, yeah.
1:12
You're connected.
1:15
That's all we need to do here.
1:16
Now, onConnectionSuspended, again, the
listener, whatever's displaying the UI,
1:18
doesn't really matter if it's suspended,
or failed, or whatnot.
1:22
It, all that matters
is it's not available.
1:26
So we're just gonna call
through to onDisconnected.
1:28
And the final one here
is onConnectionFailed.
1:32
And this is gonna happen if
the connection wasn't successful.
1:37
It failed initially, but this method's
1:42
really nice because it returns back to
us this ConnectionResult object, and
1:45
this ConnectionResult object has
a method on it called hasResolution.
1:49
So again, ConnectionResult is part
of Google Play services library and
1:54
it has this method, hasResolution, so if
there is resolution, we wanna go ahead and
2:00
try to resolve that.
2:05
So let's try to resolve this.
2:06
ConnectionResult.
2:09
And we're gonna call
startResolutionForResult.
2:10
We need to pass in our activity.
2:14
And, again,
we need to pass in a unique request code.
2:16
And earlier I said I created one
called REQUEST_CODE_RESOLUTION.
2:19
It had a unique value that was
different than my availability one.
2:24
And if we hover over this,
we see it's giving us the red line.
2:28
You're gonna see the unhandled
exception is of type
2:31
IntentSender.SendIntentException.
2:34
So, remember whenever you call
to startActivityForResult,
2:37
you're passing it in intent.
2:41
So, if this intent failed to send,
we need to handle that case.
2:43
So let's add a catch block,
and go ahead and
2:47
handle this by supplying it
the SendIntentExceptio here.
2:50
And that red error is gonna go away.
2:57
And what we wanna do here is we're
actually gonna call back to connect,
3:02
and you might think that's
a little bit weird, but
3:06
what we're doing here is,
multiple errors might be coming in, and
3:08
some of them might resolve and
some of them might not.
3:12
But if one comes in and is resolved, but
then another comes in and is failed, well,
3:15
maybe we can go back and try to connect
again and take a different path that time.
3:20
So maybe the first one,
Google Play services weren't available,
3:25
then they were available, but
then the user wasn't authorized your app.
3:28
So in any case,
we're just gonna try to connect again.
3:32
That might help resolve this resolu,
this error and resolution.
3:36
So what we want to do is try to connect.
3:40
Finally we're gonna come to a case where,
you know what, there is no resolution.
3:43
We've come across too many errors.
3:48
If all else fails,
we will finally just wanna say, all right,
3:50
listener, you're disconnected.
3:54
We couldn't resolve the errors.
3:57
We're just gonna go ahead an operate
in a state where Google Play services
4:00
isn't available right now.
4:03
And that's okay.
4:05
Maybe some other time when
the device is in a different state,
4:06
we'll be able to connect again,
but right now it's not available,
4:09
so just display the UI as
if it weren't available.
4:12
Now we need to remember that we called
multiple times these methods that
4:15
are calling through to
startActivityForResult.
4:20
And that means that they're gonna come
back in onActivityResult of our activity,
4:23
so we need a way to handle and
check what's actually coming back.
4:28
So let's go ahead and add a method, and
4:32
we're gonna call it handleActivityResult.
4:36
And what we wanna do is just take in
the same data that onActivityResult takes
4:40
as well, so we're gonna take in
the request code and the result code,
4:46
and that intent data that comes
back with onActivityResult.
4:51
Now what we wanna do is just check,
okay, well if
4:58
it was one of our codes that we sent,
we're gonna check the request codes here.
5:01
So if the request code
was either the resolution
5:05
or the request code was our availability,
5:11
okay, that means we're
gonna handle these cases.
5:16
And in either case, what we're
checking is if the result was okay.
5:20
So Activity, we have RESULT_OK.
5:27
And if it was okay,
that means some error is resolved.
5:31
Okay, let's go ahead and
try to connect again.
5:33
Otherwise, if it wasn't okay, what we're
just gonna do is say, all right, listener,
5:37
you're disconnected.
5:41
Again, we tried to resolve the error,
but it was not available to resolve.
5:42
Google Play services wasn't available or
is disabled, or for some other reason.
5:48
So again, we're just gonna fall
back to saying disconnected.
5:52
Let's go ahead and import that.
5:55
Great, now we've handled all
the edge cases for connecting and
5:59
disconnecting with Google Play services.
6:02
Let's take a break, and when we come back,
6:04
we can integrate our
helper with our UI code.
6:06
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up