Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player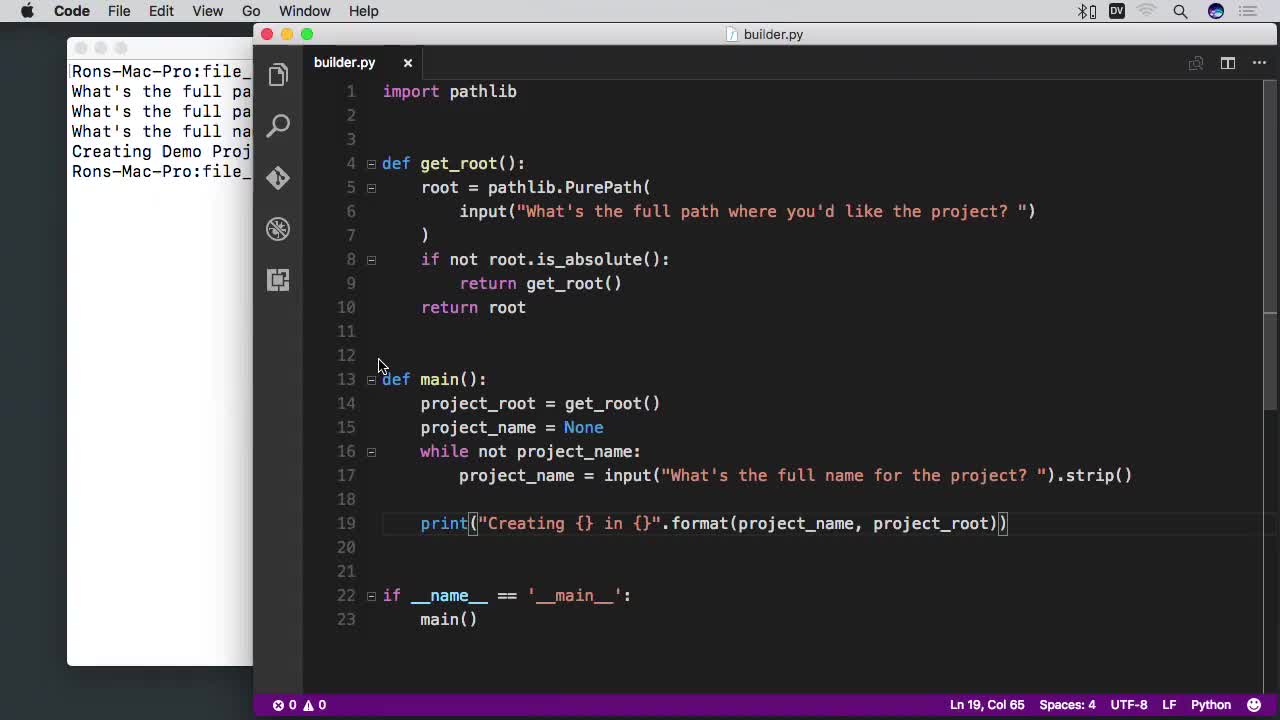
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Let's build out the directory structure of our project.
This video doesn't have any notes.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Hanwen Zhang
20,084 Points1 Answer
-
Peter Kolawa
36,765 Points7 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
Since the directory checking is probably
going to be more than just a step or
0:00
two let's put that into
a new function as well.
0:03
If the directory exists, we want to
make sure that they want to delete it
0:07
before we do that, and
we want to dump our stuff into it.
0:10
So they give us a directory,
we check to see if it's good or not.
0:13
If it exists we ask hey, do you want me
to delete this, and they will say yes or
0:17
no, and then we will act accordingly.
0:21
So I'm gonna call this check_delete_root
and it's gonna take a root, because I'm
0:25
gonna check to see if the root exists and
then whether or not to delete the root.
0:30
And I need to inboard a couple of things.
0:35
So first I'm going to import disutils.utl.
0:37
Oops, from that, import strtobool.
0:42
This is a really handy function
that we'll talk about in a second.
0:50
And then we're gonna import sys.
0:52
All right, so inside check delete root,
let's say if os.path.exists for
0:56
the root, then we're gonna print,
path already exists.
1:03
And then,
let's try delete equals strtobool
1:09
input delete existing files, directories.
1:16
And we'll encourage them to give us yes or
no.
1:23
strtobool is really cool,
so a really great function.
1:27
It allows users to give you
things like Y or yes or true.
1:30
And those are considered
to be valid yes answers.
1:35
And if they give you like n or no,
then it's considered a negative answer.
1:40
I think we might actually
need lower on that.
1:44
Let's just have lower in there anyway.
1:46
Okay.
But if there is a ValueError, so
1:50
they give us something that couldn't
be turned into a yes or no,
1:54
then let's just return a call to
check_delete_root, with the root.
1:58
So just start them over again.
2:03
Otherwise though,
if the delete came out as being true,
2:05
then let's try to remove
those directories.
2:09
And if we get an OSError which that
should be de-indented over there.
2:15
Then let's print, couldn't remove
the thing, please delete it yourself.
2:23
And we want to format that with (root).
2:33
If we could remove those though,
then let's say,
2:38
print deleted and
we'll format that with root.
2:43
And then, I'm gonna make the function
return none, no matter what.
2:48
You don't have to do this.
2:51
It would return none already on its own.
2:53
I just like to have a return there.
2:56
So, let's go over this again.
2:59
So first we're gonna check
to see if it exists.
3:00
If it does, then we tell the user,
and we say hey,
3:02
do you want us to delete this or not?
3:06
They will say yes, no, whatever,
we're gonna have those a boolean.
3:08
If that fails,
we're gonna send them right back,.
3:12
But if it doesn't fail, then we're gonna
check that boolean to see if it's true or
3:14
if it's false.
3:18
If it's true, and we're gonna
try to remove those directories.
3:18
If we can remove them,
then we're gonna say cool, hey,
3:22
we deleted this, and we're gonna go on.
3:24
If we couldn't removed them, then
we're gonna say we can't remove these,
3:27
you need to fix it yourself.
3:29
That's gonna be like,
if there's already files in there, or
3:31
if there's already other directories
in there, that we're gonna be told,
3:33
hey, you can't, we can't delete this.
3:35
Alright.
3:38
So nice.
3:39
So now, let's use this.
3:40
Actually, let's just take out that return.
3:43
We don't need that at all.
3:44
That's just extra code that we don't need.
3:47
Will you stop asking me about that?
3:48
So let's go ahead and
do [LAUGH] this right here.
3:50
We'll say, check_delete_root for
the project_root.
3:53
And we'll do this before the project name.
3:58
So that it will do that before we
even bother asking for a name.
4:00
No reason asking them for
a name if we don't have to.
4:05
So, now, let's see about getting
a safe version of the project name.
4:08
Now, I know most OS's can handle spaces
in files, and directory names now a day.
4:11
But, I'd much rather have something
predictable, and python friendly.
4:15
So, let's, more or less,
Rip off Django's Slugify function.
4:19
I need to import a couple of things first,
so I need to import RE which is
4:23
the regular expressions module,
and I need to import unicode data.
4:27
So let's make a function, I'll just make
this here at the top because it doesn't
4:33
really belong to anything else,
and we're gonna call this slugify.
4:37
That's gonna take some sort of string.
4:41
So, like I said,
this is very heavily borrowed from Django.
4:44
So thank you Django.
4:46
So first, I'm gonna make a variable and
name the string,
4:48
which is gonna be unicodedata.
4:51
.normalize.
4:53
We're gonna normalize this
along NFKC rules, and
4:56
we're gonna do that to the string.
4:59
So what this will do is this will
strip out unicode characters and
5:01
replace them with an ASCII equivalent.
5:04
So then, we're gonna say that
we want the string to be
5:08
substituted using a regular expression.
5:11
Anything that is not a word character or
a space, we want to just get rid of.
5:13
And then, we want to strip that and
lowercase that.
5:21
And then,
finally we're gonna return re.sub,
5:26
anything that is an underscore, a hyphen
or a space, a white space character.
5:30
Where there's one or more of those,
5:34
we're gonna turn into a single
underscore in the string.
5:36
That's it.
5:41
Okay, so the Unicode data library gives us
information about the characters in our
5:43
Unicode strings.
5:47
We'll need that to make sure our
strings are safe for reuse, and
5:48
we're gonna use the normalize method here,
like I said,
5:50
to change the Unicode characters
into ASCII characters.
5:53
We're gonna take anything that isn't
a Unicode word character, or a whitespace.
5:55
Replace it with nothing.
6:00
Strip and lowercase the string.
6:02
Then replace any underscores,
hyphens or spaces,
6:03
especially where we have more than
one of them into a single underscore.
6:06
And then,
we're gonna return that resulting string.
6:10
Okay, add one more thing that we need.
6:12
We need a list of directories that
we're gonna use for our project.
6:15
So let's have project_slug.
6:20
Slash, and then let's copy this.
6:23
And one, two, three, four, five, six.
6:27
I need six of these.
6:30
There's one, two, three, four, five, six.
6:31
So, first we need to have static and
then we need to have static/image.
6:35
Static/JavaScript, static/css,
6:41
and templates.
6:48
So these are all of our directories.
6:51
So now, we're going to want a directory
that's using b project slug.
6:53
Inside of that, we're gonna have
a static directory with image css and
6:56
JavaScript directories.
7:00
We also want to have a template directory
inside the project slug directory.
7:02
You can probably guess, I'm planning on
using format on these directory names.
7:05
So let's make a function that will
make all of these directories.
7:09
So we'll just call it create_dirs.
7:16
And it's gonna take the root and
it's gonna take a slug.
7:20
So we're gonna try os.makedirs the root,
whatever the root is.
7:25
But if there's an OSError on that,
then we're gonna
7:29
print "Couldn't create the project
root at ()." that place.
7:34
And then, we're gonna call .format and
pass in the root.
7:41
And then,
we're gonna immediately call sys.exit() so
7:47
that it just stops executing the program.
7:50
If everything's cool, then for
dir in DIRS, we're going to try os.mkdir,
7:54
os.path.join root, and
then whatever dir we're currently on.
8:01
But we're gonna format
that where project_slug
8:07
is equal to the slug
that's been passed in.
8:10
And if there's a file exists error,
we're just gonna pass.
8:14
Now this might be a place where we
might just wanna fail, just quit.
8:21
We should probably stop the program
here and tell them what failed, but
8:24
I'm gonna leave that up to you to add.
8:27
So let's see if this works.
8:30
We're gonna get the project name, after we
have that project name, let's go ahead and
8:31
make the project slug.
8:35
So, we'll do project_slug =
slugify(project_name) and
8:37
then, let's go ahead and call creators.
8:44
And, we're going to pass
in the project root.
8:47
And, the project slug.
8:49
Lowercase s.
8:52
Okay, let's try this again.
8:54
So I'm gonna do this, at
Users/Kenneth/Projects/file_systems/demo.
8:58
Os is not defined,
did we forget to import os?
9:08
We did.
9:12
So, O and then P.
9:14
So we'll do import OS over in that again.
9:15
And again
Users/Kenneth/Projects/file_systems/demo/.
9:19
And I'm gonna call this Demo Project.
9:27
I got a bad character range.
9:31
I think I know what's wrong with that.
9:33
There should be a backslash right there.
9:35
Be great if I didn't have to type
out this whole thing wouldn't it?
9:39
Users/Kenneth/Projects/file_systems/demo
DemoProject.
9:42
And it's gonna create
DemoProject in there.
9:53
Let's go over here and look at Finder,
we have demo, and we have demoproject.
9:55
And notice, it's all lowercase even though
I had uppercase characters in there.
9:59
If I look inside here, I have a static
directory with those directories in them.
10:01
And I have a templates directory
which will have nothing in it.
10:05
So that's pretty awesome,
great job Python.
10:09
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up